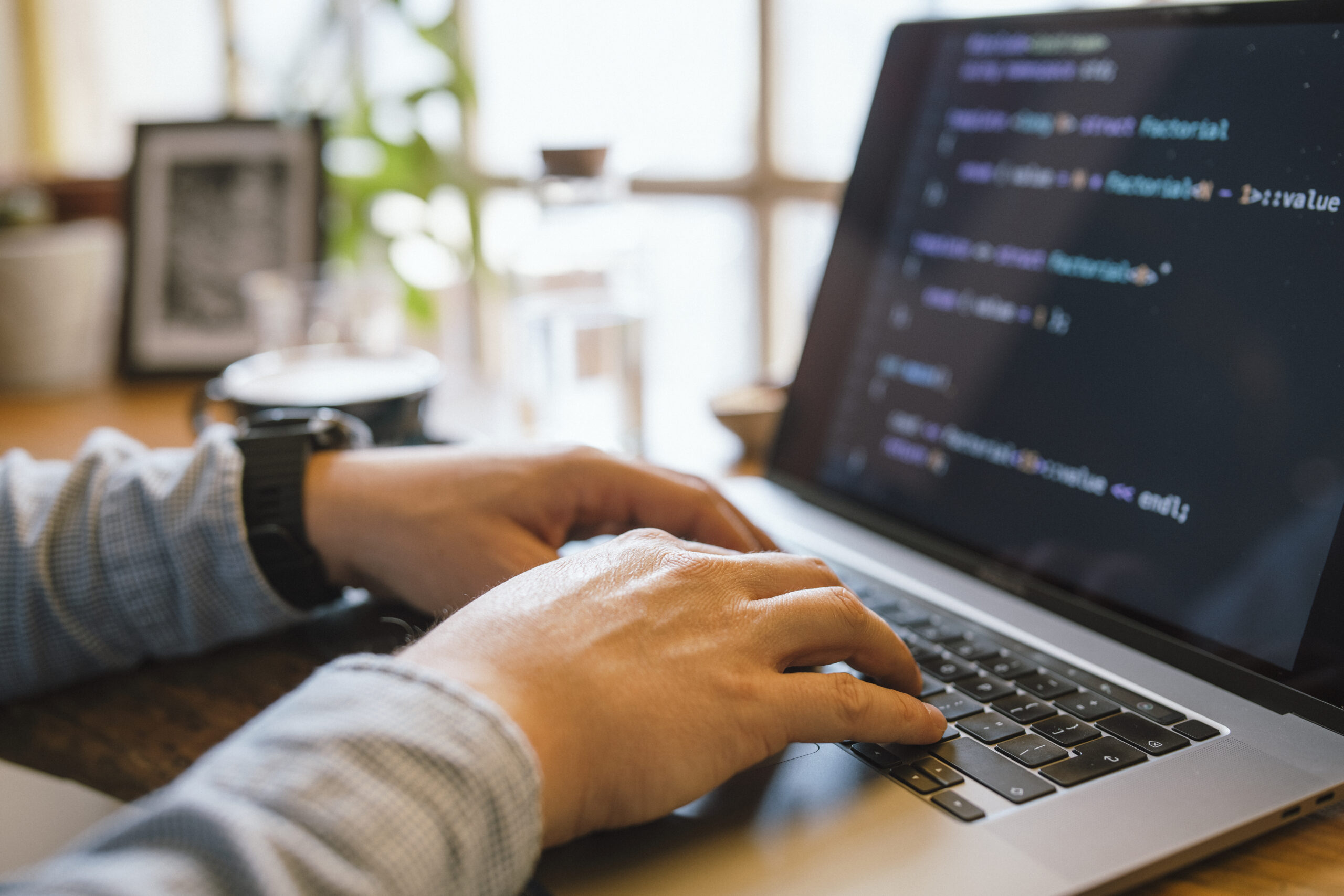
Debugging is one of the most crucial — nonetheless often ignored — capabilities in a very developer’s toolkit. It isn't really pretty much correcting damaged code; it’s about comprehending how and why issues go Improper, and Finding out to Consider methodically to resolve troubles successfully. No matter if you are a rookie or maybe a seasoned developer, sharpening your debugging techniques can help save hrs of stress and substantially increase your productiveness. Listed below are numerous techniques to assist developers amount up their debugging activity by me, Gustavo Woltmann.
Grasp Your Equipment
One of many quickest means builders can elevate their debugging capabilities is by mastering the resources they use each day. While crafting code is just one Section of advancement, knowing ways to communicate with it efficiently throughout execution is Similarly critical. Contemporary enhancement environments appear equipped with impressive debugging capabilities — but lots of builders only scratch the surface area of what these tools can do.
Just take, as an example, an Integrated Progress Ecosystem (IDE) like Visible Studio Code, IntelliJ, or Eclipse. These equipment permit you to established breakpoints, inspect the worth of variables at runtime, stage as a result of code line by line, and in some cases modify code within the fly. When made use of accurately, they let you notice specifically how your code behaves all through execution, that's invaluable for monitoring down elusive bugs.
Browser developer applications, for example Chrome DevTools, are indispensable for front-conclude builders. They let you inspect the DOM, monitor network requests, perspective genuine-time general performance metrics, and debug JavaScript inside the browser. Mastering the console, resources, and network tabs can switch frustrating UI troubles into manageable jobs.
For backend or system-degree builders, resources like GDB (GNU Debugger), Valgrind, or LLDB present deep Manage about operating procedures and memory administration. Understanding these instruments may have a steeper Finding out curve but pays off when debugging general performance problems, memory leaks, or segmentation faults.
Outside of your IDE or debugger, become cozy with Model Command techniques like Git to be aware of code history, locate the exact minute bugs were being released, and isolate problematic modifications.
Eventually, mastering your equipment implies heading over and above default options and shortcuts — it’s about establishing an personal familiarity with your progress ecosystem so that when issues arise, you’re not lost at midnight. The better you realize your resources, the more time you are able to invest solving the actual problem rather than fumbling through the procedure.
Reproduce the condition
One of the more important — and sometimes neglected — measures in successful debugging is reproducing the issue. Prior to leaping in the code or generating guesses, developers require to produce a reliable natural environment or situation exactly where the bug reliably seems. Devoid of reproducibility, repairing a bug gets to be a game of probability, typically leading to squandered time and fragile code alterations.
The first step in reproducing a dilemma is collecting as much context as is possible. Question concerns like: What steps resulted in The difficulty? Which setting was it in — improvement, staging, or output? Are there any logs, screenshots, or error messages? The more depth you've, the a lot easier it gets to isolate the exact ailments below which the bug takes place.
After you’ve collected plenty of details, seek to recreate the challenge in your neighborhood surroundings. This may suggest inputting the same knowledge, simulating similar consumer interactions, or mimicking procedure states. If the issue seems intermittently, consider composing automatic tests that replicate the edge scenarios or state transitions concerned. These checks not only support expose the condition but additionally protect against regressions in the future.
At times, The difficulty may be surroundings-precise — it'd occur only on specified functioning systems, browsers, or below distinct configurations. Applying tools like virtual devices, containerization (e.g., Docker), or cross-browser screening platforms is often instrumental in replicating these types of bugs.
Reproducing the issue isn’t only a phase — it’s a way of thinking. It necessitates patience, observation, along with a methodical strategy. But as soon as you can continually recreate the bug, you might be already midway to correcting it. With a reproducible scenario, You should use your debugging resources a lot more efficiently, examination likely fixes safely and securely, and converse far more Plainly using your crew or end users. It turns an abstract grievance into a concrete challenge — Which’s where by developers prosper.
Examine and Fully grasp the Mistake Messages
Error messages are frequently the most precious clues a developer has when some thing goes Incorrect. Rather than looking at them as disheartening interruptions, builders must find out to treat mistake messages as immediate communications from your method. They often show you just what exactly occurred, exactly where it happened, and from time to time even why it occurred — if you know how to interpret them.
Get started by looking at the message carefully As well as in total. Many builders, especially when less than time force, glance at the main line and quickly begin earning assumptions. But further in the mistake stack or logs might lie the legitimate root lead to. Don’t just duplicate and paste error messages into search engines like google — browse and recognize them first.
Split the error down into areas. Is it a syntax error, a runtime exception, or simply a logic error? Will it stage to a certain file and line quantity? What module or purpose triggered it? These inquiries can guide your investigation and position you towards the accountable code.
It’s also handy to know the terminology with the programming language or framework you’re utilizing. Error messages in languages like Python, JavaScript, or Java normally stick to predictable designs, and learning to recognize these can considerably speed up your debugging method.
Some faults are vague or generic, As well as in Those people circumstances, it’s important to look at the context by which the error transpired. Look at related log entries, input values, and recent improvements in the codebase.
Don’t neglect compiler or linter warnings both. These generally precede larger sized problems and provide hints about likely bugs.
In the long run, mistake messages are not your enemies—they’re your guides. Studying to interpret them appropriately turns chaos into clarity, supporting you pinpoint challenges faster, decrease debugging time, and become a much more effective and assured developer.
Use Logging Properly
Logging is The most highly effective applications inside of a developer’s debugging toolkit. When used effectively, it provides real-time insights into how an software behaves, encouraging you understand what’s going on underneath the hood without having to pause execution or move in the code line by line.
A fantastic logging tactic commences with figuring out what to log and at what stage. Widespread logging amounts contain DEBUG, Information, WARN, Mistake, and Lethal. Use DEBUG for thorough diagnostic data in the course of advancement, Information for general situations (like thriving start out-ups), WARN for possible issues that don’t crack the appliance, ERROR for actual complications, and Deadly once the system can’t go on.
Keep away from flooding your logs with extreme or irrelevant data. Far too much logging can obscure significant messages and slow down your procedure. Center on crucial events, point out adjustments, enter/output values, and significant choice points as part of your code.
Format your log messages Evidently and constantly. Include context, for instance timestamps, request IDs, and performance names, so it’s easier to trace difficulties in distributed devices or multi-threaded environments. Structured logging (e.g., JSON logs) could make it even much easier to parse and filter logs programmatically.
In the course of debugging, logs Permit you to monitor how variables evolve, what ailments are satisfied, and what branches of logic are executed—all devoid of halting the program. They’re In particular beneficial in generation environments exactly where stepping by code isn’t possible.
Moreover, use logging frameworks and tools (like Log4j, Winston, or Python’s logging module) that help log rotation, filtering, and integration with checking dashboards.
Ultimately, smart logging is about equilibrium and clarity. Having a very well-thought-out Gustavo Woltmann coding logging strategy, you could reduce the time it requires to identify issues, obtain further visibility into your purposes, and improve the All round maintainability and dependability within your code.
Think Just like a Detective
Debugging is not simply a complex task—it's a type of investigation. To properly detect and fix bugs, developers need to technique the procedure similar to a detective resolving a secret. This state of mind aids break down intricate difficulties into workable pieces and follow clues logically to uncover the root trigger.
Commence by collecting evidence. Consider the indicators of the challenge: mistake messages, incorrect output, or effectiveness difficulties. Identical to a detective surveys against the law scene, accumulate just as much suitable facts as you may devoid of leaping to conclusions. Use logs, take a look at situations, and consumer studies to piece with each other a clear picture of what’s going on.
Future, variety hypotheses. Check with on your own: What could be producing this habits? Have any alterations just lately been created towards the codebase? Has this problem happened in advance of underneath equivalent situations? The goal should be to slim down prospects and determine opportunity culprits.
Then, take a look at your theories systematically. Try and recreate the issue in a very controlled atmosphere. For those who suspect a certain perform or component, isolate it and validate if the issue persists. Similar to a detective conducting interviews, talk to your code issues and Allow the effects direct you closer to the reality.
Pay out close attention to compact information. Bugs usually hide from the minimum expected sites—just like a missing semicolon, an off-by-a person error, or even a race condition. Be extensive and patient, resisting the urge to patch The problem with out thoroughly knowing it. Non permanent fixes could cover the real dilemma, just for it to resurface later.
And lastly, keep notes on Whatever you tried using and realized. Equally as detectives log their investigations, documenting your debugging procedure can help save time for future concerns and assistance Other people fully grasp your reasoning.
By thinking like a detective, developers can sharpen their analytical techniques, approach difficulties methodically, and develop into more practical at uncovering hidden concerns in advanced units.
Create Exams
Producing checks is among the simplest methods to increase your debugging techniques and In general development efficiency. Exams not merely support capture bugs early but will also function a security Web that offers you confidence when creating modifications in your codebase. A effectively-analyzed software is much easier to debug mainly because it helps you to pinpoint exactly where and when a problem occurs.
Get started with device assessments, which center on particular person capabilities or modules. These smaller, isolated assessments can speedily reveal regardless of whether a particular piece of logic is working as expected. When a exam fails, you straight away know wherever to glance, drastically minimizing time spent debugging. Device assessments are Specially beneficial for catching regression bugs—problems that reappear after Beforehand currently being mounted.
Subsequent, combine integration assessments and stop-to-end checks into your workflow. These support make certain that numerous aspects of your application function alongside one another efficiently. They’re especially useful for catching bugs that come about in sophisticated systems with many elements or products and services interacting. If a thing breaks, your tests can inform you which Portion of the pipeline unsuccessful and beneath what conditions.
Composing tests also forces you to definitely think critically regarding your code. To test a attribute correctly, you require to comprehend its inputs, envisioned outputs, and edge circumstances. This volume of knowing The natural way qualified prospects to raised code structure and less bugs.
When debugging a difficulty, creating a failing take a look at that reproduces the bug may be a strong starting point. Once the examination fails continuously, you'll be able to deal with fixing the bug and look at your exam pass when The problem is solved. This solution ensures that precisely the same bug doesn’t return Down the road.
In brief, composing checks turns debugging from the irritating guessing match right into a structured and predictable system—assisting you catch far more bugs, a lot quicker and more reliably.
Get Breaks
When debugging a difficult challenge, it’s quick to be immersed in the problem—looking at your display for hrs, striving Option just after solution. But Probably the most underrated debugging resources is just stepping away. Getting breaks can help you reset your head, cut down irritation, and infrequently see The difficulty from the new standpoint.
If you're far too near the code for much too extensive, cognitive exhaustion sets in. You may perhaps start out overlooking noticeable glitches or misreading code you wrote just hrs previously. On this state, your brain becomes less economical at trouble-fixing. A short walk, a espresso crack, as well as switching to a distinct activity for 10–quarter-hour can refresh your concentration. A lot of developers report finding the foundation of a challenge once they've taken time to disconnect, permitting their subconscious get the job done while in the track record.
Breaks also help reduce burnout, Specially in the course of lengthier debugging classes. Sitting in front of a display screen, mentally stuck, is not only unproductive and also draining. Stepping away allows you to return with renewed Electricity as well as a clearer mindset. You may perhaps out of the blue discover a lacking semicolon, a logic flaw, or perhaps a misplaced variable that eluded you right before.
In case you’re stuck, a fantastic rule of thumb will be to set a timer—debug actively for forty five–60 minutes, then take a five–10 moment break. Use that point to move all around, extend, or do one thing unrelated to code. It may well truly feel counterintuitive, especially beneath limited deadlines, nevertheless it basically results in a lot quicker and more effective debugging In the long term.
In short, using breaks is not really a sign of weak point—it’s a sensible technique. It offers your Mind space to breathe, enhances your point of view, and helps you stay away from the tunnel eyesight That always blocks your progress. Debugging is actually a psychological puzzle, and relaxation is an element of solving it.
Understand From Each individual Bug
Each bug you come across is a lot more than simply a temporary setback—It really is a chance to improve as a developer. Regardless of whether it’s a syntax mistake, a logic flaw, or maybe a deep architectural issue, each one can educate you anything precious for those who make an effort to reflect and examine what went Mistaken.
Start out by inquiring yourself a few important queries after the bug is solved: What induced it? Why did it go unnoticed? Could it are caught before with superior tactics like device tests, code opinions, or logging? The responses generally expose blind places with your workflow or comprehension and allow you to Make more robust coding practices going ahead.
Documenting bugs can even be a fantastic practice. Hold a developer journal or sustain a log where you note down bugs you’ve encountered, the way you solved them, and Anything you figured out. After some time, you’ll start to see patterns—recurring issues or popular faults—you can proactively keep away from.
In group environments, sharing what you've learned from the bug using your peers is usually In particular strong. Regardless of whether it’s through a Slack information, a short write-up, or A fast information-sharing session, helping Many others stay away from the exact same difficulty boosts crew efficiency and cultivates a more robust Understanding society.
Far more importantly, viewing bugs as lessons shifts your way of thinking from disappointment to curiosity. Instead of dreading bugs, you’ll start out appreciating them as important aspects of your advancement journey. After all, many of the very best builders aren't those who write best code, but those who continually learn from their problems.
Eventually, Each and every bug you take care of adds a different layer to your ability established. So next time you squash a bug, take a minute to reflect—you’ll arrive absent a smarter, extra capable developer on account of it.
Summary
Enhancing your debugging capabilities usually takes time, apply, and endurance — though the payoff is huge. It can make you a far more efficient, confident, and capable developer. The subsequent time you might be knee-deep in a mysterious bug, bear in mind: debugging isn’t a chore — it’s a chance to be improved at Everything you do.